Use MongoDB with Lucee
In my attempt to use MongoDB with Lucee I used the following extension:
MongoDB Datasource and Cache
// Connecting to mongodb and database
db = MongoDBConnect("collection", "mongodb://user:pass@host:port/collection?authSource=admin");
// Check if collection exists else create one
if(!db.getCollectionNames().find("data")){
db.createCollection(
"data",
{
timeseries: {
timeField: "timestamp",
metaField: "data",
granularity: "hours"
},
expireAfterSeconds: 86400
}
)
}
// Create a random number and insert to collection
rndmNumber = ceiling(rand() * 10);
db["logs"].insert( { item: "card", qty: rndmNumber } );
// Get amount of all documents in the collection
fullCollection = db.getCollection("collection").count();
dump(fullCollection);
// Query data
find = db["logs"].find( { qty: { '$gt': 4 } } ).sort( { qty: 1 } ).limit( 5 ).toArray();
findOne = db["logs"].findOne({ qty: 10});
dump(findOne);
dump(find);
To pass in collection options, I had to use the Java Libraries directly. In the following example, I created a collection with validation options. This prevents data that does not match the schema from being written to the collection. The extension above includes all the additional needed Java libraries.
connectionString = "mongodb://#variables.mongoUsername#:#variables.mongoPassword#@#variables.mongoHost#:#variables.mongoPort#/demo?authSource=admin";
mongoConnectionString = loadClass( "com.mongodb.ConnectionString" )
.init( connectionString )
;
mongoClient = loadClass( "com.mongodb.client.MongoClients" )
.create( mongoConnectionString )
;
mongoCreateCollectionOptions = loadClass( "com.mongodb.client.model.CreateCollectionOptions" );
mongoValidator = loadClass( "com.mongodb.client.model.ValidationOptions" );
db = mongoClient.getDatabase(mongoConnectionString.getDatabase());
optionValidation = mongoValidator.validator(
toBson(
[
"$jsonSchema": {
"bsonType": 'object',
"required": [ 'severity', 'operation', 'data' ],
"properties": {
"severity": {
"bsonType": 'double',
"description": 'Must be a double and is required!'
},
"operation": {
"bsonType": 'string',
"description": 'Must be a string and is required!'
},
"data": {
"bsonType": 'object',
"required": [ 'message' ],
"properties": {
"message": {
"bsonType": 'string',
"description": 'Must be a string and is required!'
}
}
}
}
}
]
)
);
dump(mongoValidator.getValidator());
db.createCollection("logs", mongoCreateCollectionOptions.validationOptions(optionValidation));
// Functions
// https://www.bennadel.com/blog/3905-getting-mongodb-database-and-collection-names-from-the-connection-string-in-lucee-cfml-5-3-6-61.htm
public any function loadClass( required string className ) {
return( createObject( "java", className ) );
}
public any function toBson( required struct orderedKeyValuePairs ) {
return( loadClass( "org.bson.Document" ).init( orderedKeyValuePairs ) );
}
public struct function fromBson( required any bsonDocument ) {
var unwrappedStruct = structNew( "linked" ).append( bsonDocument );
loop
index = "local.key"
item = "local.value"
struct = unwrappedStruct
{
if ( ! isNull( value ) && isStruct( value ) ) {
unwrappedStruct[ key ] = fromBson( value );
}
}
return( unwrappedStruct );
}
I released a working example on my GitHub:
GitHub - rabume/mongodb-lucee
Contribute to rabume/mongodb-lucee development by creating an account on GitHub.
References
Getting MongoDB Database And Collection Names From The Connection Stri
Ben Nadel looks at how to extract MongoDB database and collection names from an ENV-based connection string such that the document database can be consumed more opaquely in Lucee CFML 5.3.6.61.
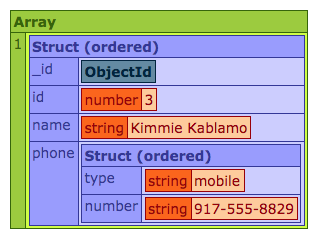